패스트 캠퍼스 강의로 "네고왕 이벤트 선착순 쿠폰 시스템" 강의를 들으면서 express + bulljs + prisma 조합으로 만들어 보았습니다.
Coupon은 쿠폰의 종류와 쿠폰의 유효기간, 발급 수량, 발급된 수량을 관리합니다.
CouponIssue는 어떤 유저가 쿠폰을 발급 받았는지를 관리합니다.
model Coupon {
id Int @id @default(autoincrement())
title String // 쿠폰명
totalQuantity Int? // 쿠폰 발급 최대 수량
issuedQuantity Int // 발급된 쿠폰 수량
issueStartDate DateTime // 발급 시작 일시
issueEndDate DateTime // 발급 종료 일시
couponIssue CouponIssue[]
}
model CouponIssue {
id Int @id @default(autoincrement())
couponId Int
coupon Coupon @relation(fields: [couponId],references: [id])
userId Int @unique
}
유저가 쿠폰을 발급 받기위해서는 쿠폰을 검증해야 합니다.
1. 발급 수량이 존재하는지 ?
2. 쿠폰의 유효기간에 속하는지 ?
3. 해당 유저가 이미 쿠폰을 발급 받았는지 ?
private validateCoupon = async (params: { couponId: number; userId }) => {
const { couponId, userId } = params;
if (await this.checkAlreadyIssued({ userId, couponId })) {
throw new Error("해당 유저는 이미 쿠폰을 발급받았습니다.");
}
if (!(await this.availableCouponDate({ couponId }))) {
throw new Error("정확하지 않은 기한입니다.");
}
if (!(await this.availableIssueQuantity({ couponId }))) {
throw new Error("모든 쿠폰이 소진되었습니다.");
}
};
private checkAlreadyIssued = async (params: {
userId: number;
couponId: number;
}): Promise<boolean> => {
const couponIssue = await this.prisma.couponIssue.findFirst({
where: {
userId: params.userId,
couponId: params.couponId,
},
});
return couponIssue !== null;
};
private availableIssueQuantity = async (params: {
couponId: number;
}): Promise<boolean> => {
const coupon = await this.findCoupon({ couponId: params.couponId });
if (coupon.totalQuantity === null) return true;
return coupon.totalQuantity > coupon.issuedQuantity;
};
private availableCouponDate = async (params: {
couponId: number;
}): Promise<boolean> => {
const coupon = await this.findCoupon({ couponId: params.couponId });
const now = new Date();
return coupon.issueStartDate < now && coupon.issueEndDate > now;
};
이후 쿠폰 검증을 통과하면 유저에게 쿠폰 발급을 하고, 쿠폰 발급 횟수를 +1 합니다.
saveCouponIssue = async (params: { userId: number; couponId: number }) => {
await this.prisma.couponIssue.create({
data: {
userId: params.userId,
couponId: params.couponId,
},
});
const coupon = await this.prisma.coupon.findFirstOrThrow({
where: {
id: params.couponId,
},
});
await this.prisma.coupon.update({
where: {
id: coupon.id,
},
data: {
issuedQuantity: coupon.issuedQuantity + 1,
},
});
};
이제 테스트를 해볼건데, 테스팅 툴로는 locust를 사용했으며 1000명까지 초당 100명씩 증가하면서 유저가 /issueCouponV1으로 요청을 보냈을 때의 테스트 입니다.
쿠폰의 최대 발급 수량(totalQuantity)은 500개 입니다. 쿠폰이 모두 발급 되었을 때 예상되는 상황은 issuedQuantity값이 500이며, CouponIssue의 row가 500개여야 합니다.
app.post("/issueCoupon", async (req: any, res: any, next) => {
try {
const couponService = new CouponIssueService(prisma);
await couponService.issueCoupon({
userId: req.body.userId,
couponId: req.body.couponId,
});
return res.status(200).json({ message: "쿠폰 발급 성공" });
} catch (err) {
return next(err);
}
});
....
issueCoupon = async (params: { userId: number; couponId: number }) => {
await this.validateCoupon({
couponId: params.couponId,
userId: params.userId,
});
await this.saveCouponIssue({
userId: params.userId,
couponId: params.couponId,
});
};
젤 아래칸은 Number Of Users
가운데칸은 Response Times(ms)
젤 위에칸은 Total Requests per Seconds, RPS
쿠폰을 발급받는과정을 보면 RPS는 500정도 나오며, DB CPU 사용량은 75%정도 됩니다.
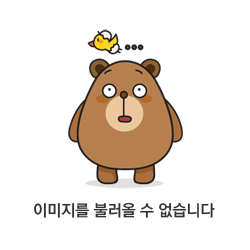

쿠폰을 모두 발급했을 때는 RPS가 올라가는 것을 볼 수 있습니다. 아무래도 CouponIssue테이블에 insert, update 하는 작업이 없어서 그런 것 같습니다. RPS가 오르면서 mysql CPU도 같이 올라가는 것을 볼 수 있습니다.
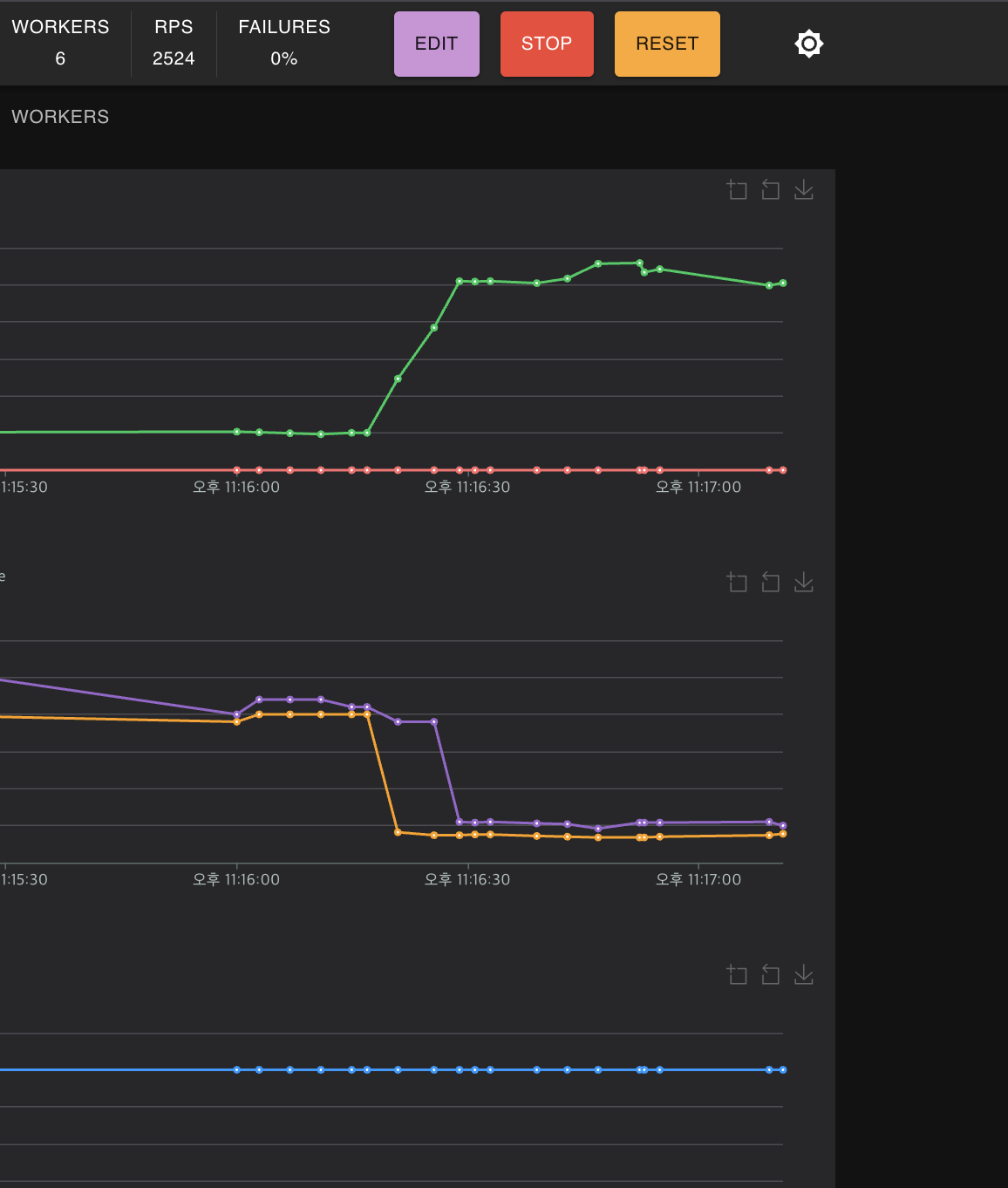

그렇다면 쿠폰은 정확히 500개가 발급되었을까요 ?
issuedQuantity는 502이며, 유저에 발급된 쿠폰은 총 84293개 입니다. 실제 발급해야 하는 500개보다 훨씬 더 많은 쿠폰이 발급되었습니다.
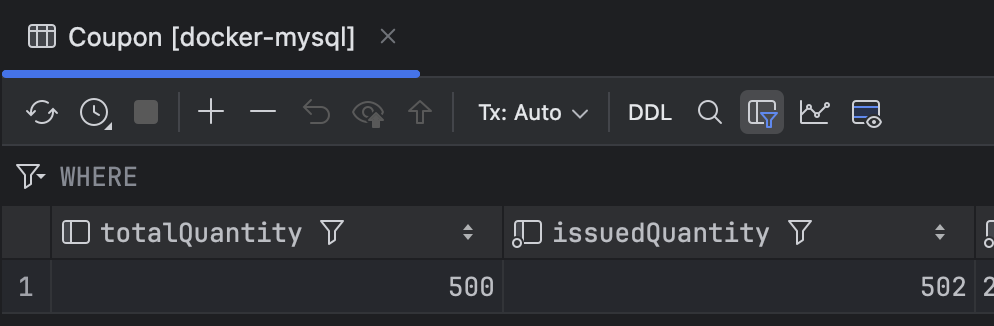
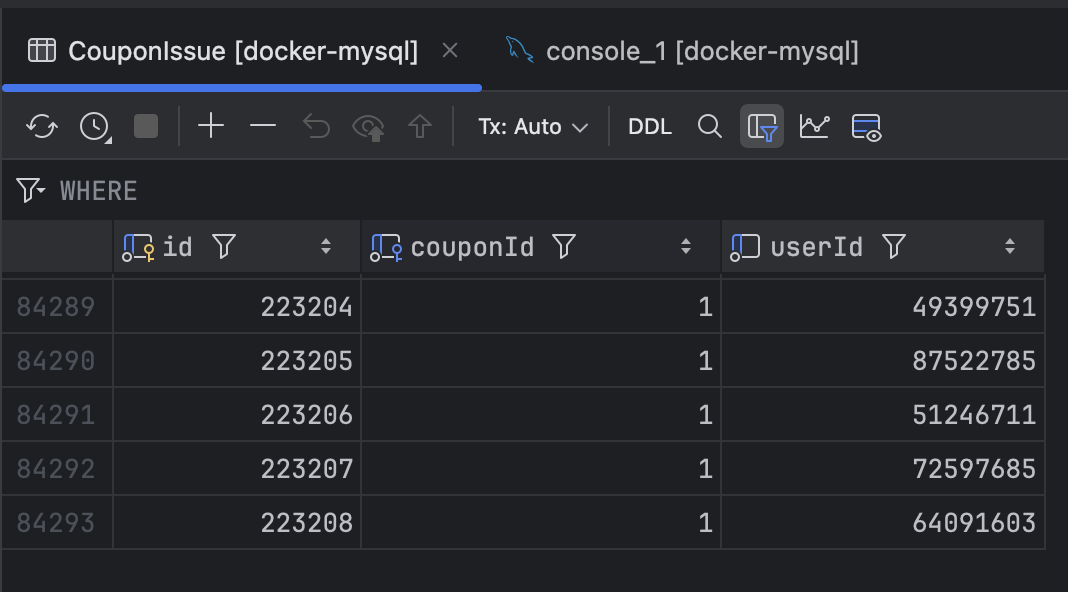
500개보다 더 많은 쿠폰이 발급된 이유는, 많은 요청이 실행되면서 로직에 Coupon의 issuedQuantity+1 하기전에 쿠폰의 잔여 수량을 검사하는 availableIssueQuantity 함수가 더 많이 실행되었기 때문이며, availableIssueQuantity 조건은 참이 되어 더 많은 쿠폰을 발급하게 되는 것입니다.
await this.prisma.coupon.update({
where: {
id: coupon.id,
},
data: {
issuedQuantity: coupon.issuedQuantity + 1,
},
});
...
private availableIssueQuantity = async (params: {
couponId: number;
}): Promise<boolean> => {
const coupon = await this.findCoupon({ couponId: params.couponId });
if (coupon.totalQuantity === null) return true;
return coupon.totalQuantity > coupon.issuedQuantity;
};
동시성 제어
이를 해결하는 방법은 쿠폰을 검증하고, 발급하는 부분을 임계영역으로 지정하여 동시성 처리를 해야 합니다. 즉, 여러 요청이 동시에 오더라도 쿠폰 검증/발급하는 부분을 순차적으로 처리하도록 해야 합니다.
이를 위해, redis-semaphore 을 사용하도록 하겠습니다.
app.post("/issueCouponV1", async (req: any, res: any, next) => {
const mutex = new Mutex(await Redis.getRedisClient(), "couponKey");
try {
await mutex.acquire();
const couponService = new CouponIssueService(prisma);
await couponService.issueCoupon({
userId: req.body.userId,
couponId: req.body.couponId,
});
return res.status(200).json({ message: "쿠폰 발급 성공" });
} catch (err) {
return next(err);
} finally {
await mutex.release();
}
});
임계영역인 issueCoupon에 진입하기 전에 key를 획득(acquire)하고 후에 반납(release)하고 있습니다.
동시성 제어를 적용한 후, RPS는 504 -> 135로 감소됐습니다. RPS가 감소되면서 mysql CPU 사용량은 자연스레 떨어졌습니다.
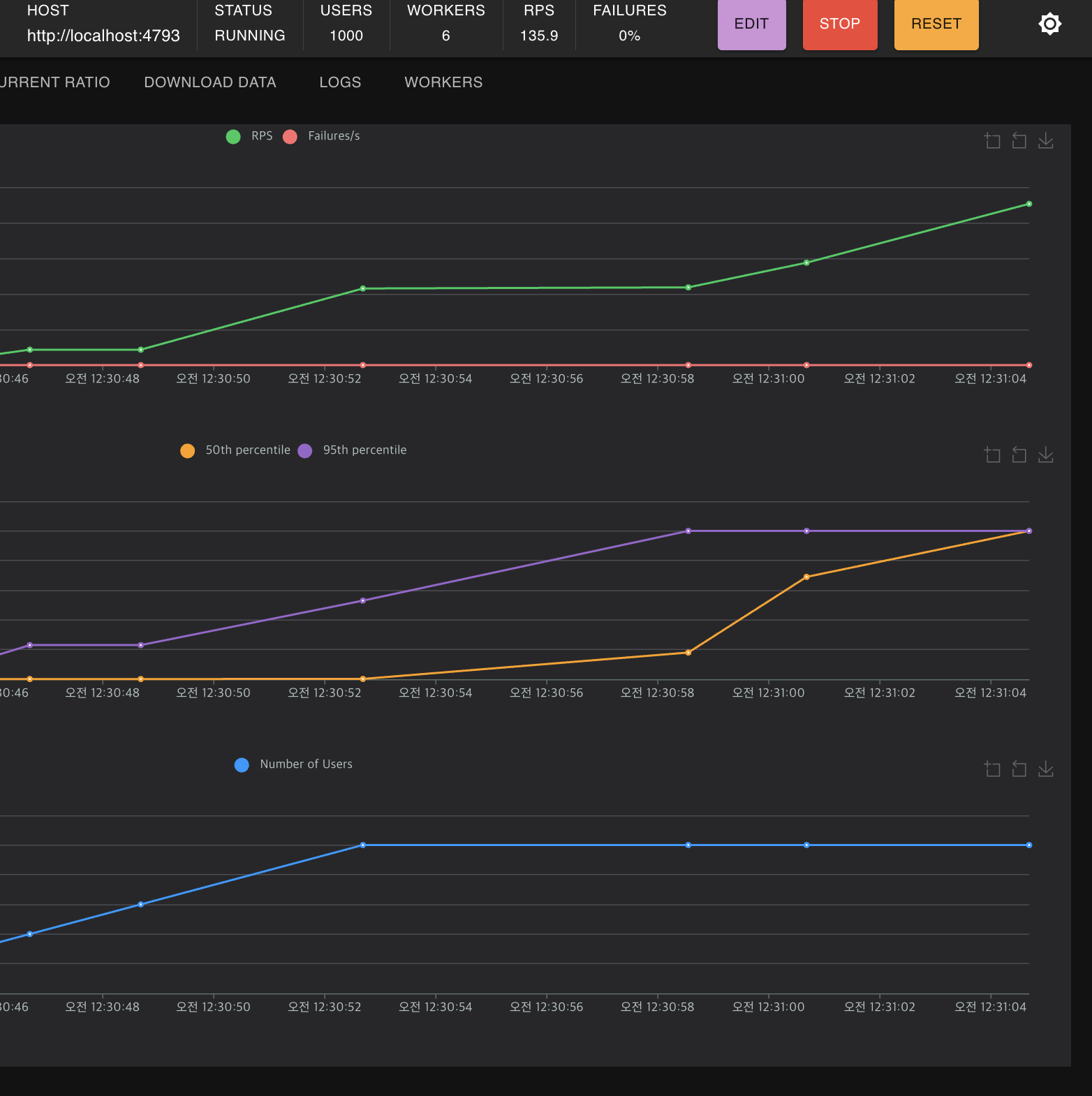

RPS는 감소되었지만, 정확히 500개의 쿠폰만 발급된 것을 확인할 수 있습니다.
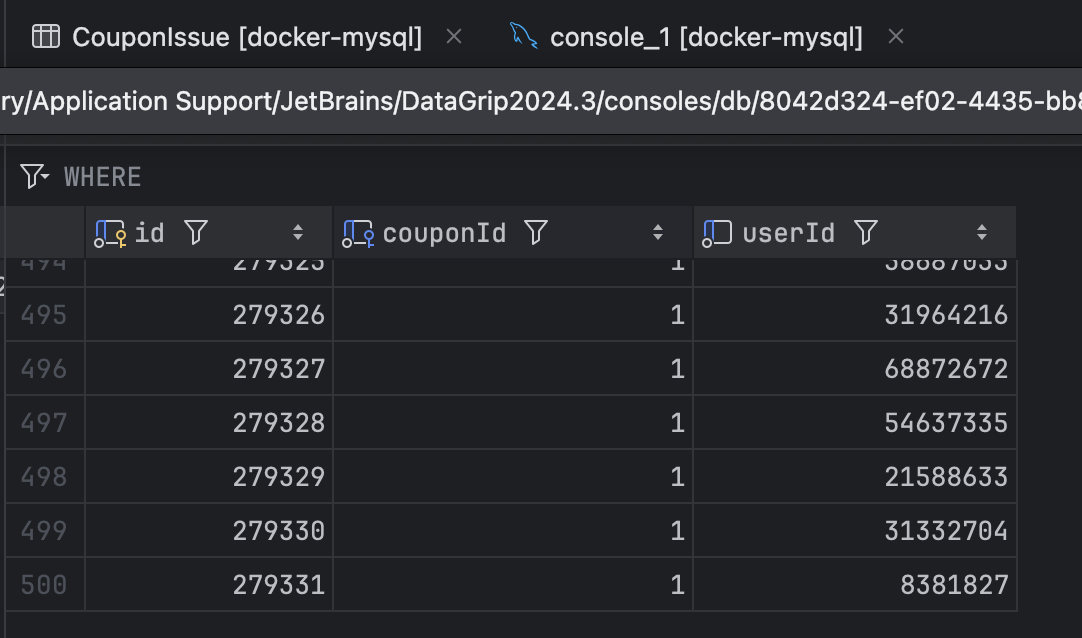
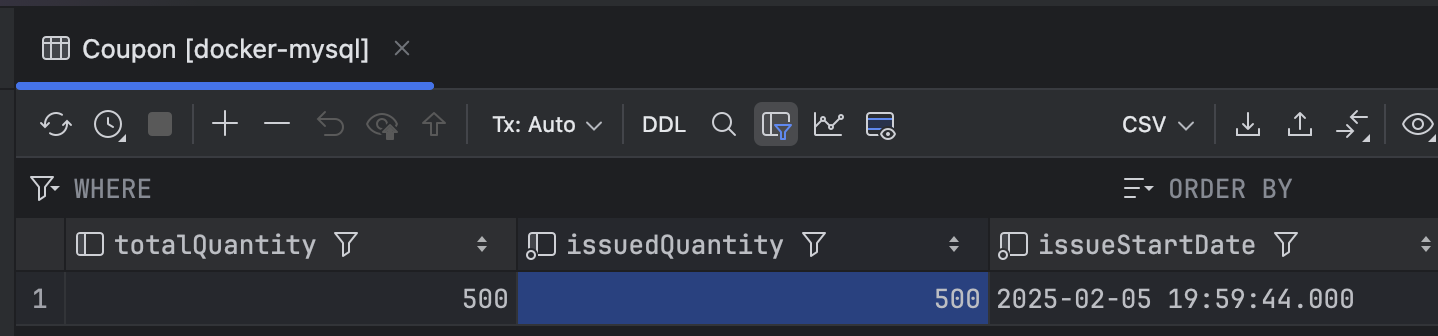
Redis, bulljs로 처리량 높히기
지금까지 만들었떤 쿠폰 발급 서버의 구조를 생각해보면, 많은 요청을 받아 정확한 쿠폰수를 발급하기 위해 임계영역을 지정하여 쿠폰을 발급하였습니다. 임계영역에서는 DB에서 쿠폰을 조회하여 검증 한 후, CouponIssue를 생성하여 DB에 삽입하고, Coupon의 issueQuantity를 +1하였습니다. 즉, 요청을 받으면 쿠폰 발급과정까지 처리해 주었습니다.
하지만, 좀 더 생각해보면 사용자는 쿠폰을 받고 바로 사용하지 않을 것이기 때문에 요청이 오면 "쿠폰을 발급 성공 여부"만 확인해주면 됩니다. 사용자에게는 쿠폰 발급 성공 여부만 응답으로 보내고, 서버에서 "쿠폰 발급할 유저 저장"한 다음에 이후 다른 서버에서 저장된 유저의 쿠폰을 발급하면 될 것입니다.
여기서 "쿠폰 발급한 유저 저장" 하는 용도로 queue를 사용할 것입니다.
쿠폰 발급 요청이 오면, 쿠폰 발급 대상자를 queue에 넣고 성공 여부를 응답으로 보냅니다. 이후 쿠폰 발급을 처리하는 서버에서는 queue에 있는 데이터를 읽어 실제 DB에 저장합니다. 여기서 queue는 bulljs를 사용하겠습니다.
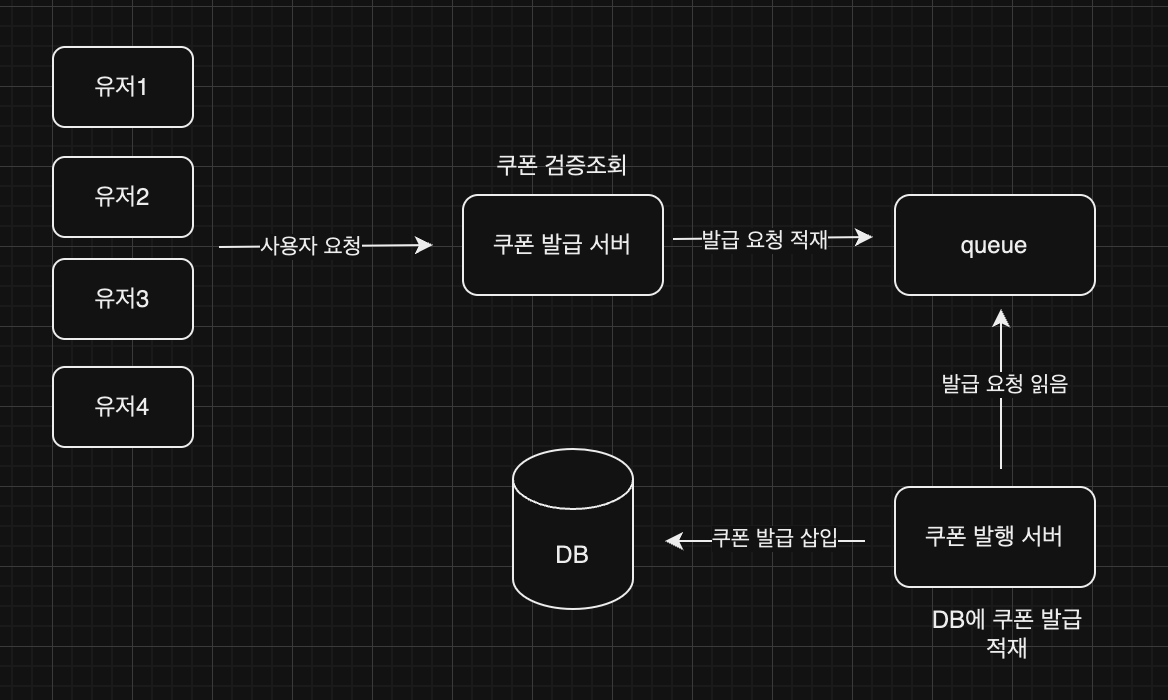
쿠폰 발급서버에서 쿠폰 발행 서버에서 유저의 쿠폰을 DB에 삽입하기 때문에, 쿠폰 발급서버에서는 쿠폰 검증만 확인하면 됩니다. 때문에 DB대신 redis를 사용하여 쿠폰 검증을 처리할 것입니다.
쿠폰 발급 서버
- 유저가 이미 쿠폰을 발급 받았는지 검증
- 쿠폰의 유효기간 검증
- 쿠폰 잔여 수량 검증
쿠폰 발행 서버
- queue에 있는 쿠폰을 가져와 DB에 삽입
쿠폰 발급 서버에서 유저가 이미 쿠폰을 발급 받았는지 검증할 수 있는 방법을 생각해보면...유저는 쿠폰을 한번만 발급받을 수 있고 언제 받았는지는 관심 없습니다. 선착순 안에 포함되는지 여부만 확인할 수 있으면 됩니다.
redis set을 사용하면, 기능을 구현할 수 있는데 set은 중복을 허용하지 않기 때문에 유저의 중복 쿠폰 발급을 막을 수 있습니다. 또한 set의 길이는 이미 쿠폰을 발급받은 유저이기 때문에, 쿠폰의 잔여수량 검증 즉 해당 유저가 현재 쿠폰 발급을 받을 수 있는지 확인할 수 있습니다.
먼저 쿠폰 정보를 Hash에 저장합니다. 이 값은 "쿠폰의 유효기간 검증"와 "쿠폰 잔여 수량 검증"에서 사용됩니다.
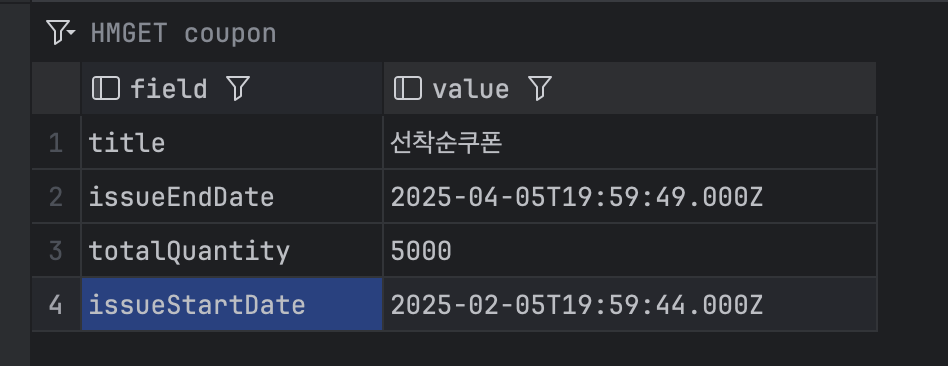
유저가 이미 쿠폰을 발급 받았는지 검증
쿠폰을 발급받은 유저 id는 set에 저장되기 때문에, 해당 유저 id가 set에 저장되어 있는지 여부로 쿠폰 발급 받았는지를 확인할 수 있습니다.
private checkAlreadyIssued = async (params: { userId: number }) => {
return await this.redis.sismember(this.couponSet, params.userId);
};
쿠폰의 유효기간 검증
moment로 해당 기간안에 쿠폰의 시작/종료 날짜가 포함되는지 검사합니다.
private availableCouponDate = async (): Promise<boolean> => {
const now = moment();
const { issueStartDate, issueEndDate } = await this.redis.hgetall("coupon");
return now.isBetween(moment(issueStartDate), moment(issueEndDate));
};
쿠폰 잔여 수량 검증
set에는 쿠폰을 발급받은 유저가 저장되어 있기 때문에 set의 크기가 쿠폰 발급 최대수량 보다 적으면 쿠폰 발급할 수 있습니다.
private availableIssueQuantity = async (): Promise<boolean> => {
const totalQuantity = await this.redis.hget("coupon", "totalQuantity");
return (await this.redis.scard(this.couponSet)) < parseInt(totalQuantity);
};
쿠폰 발급하기
쿠폰 검증을 하면 set에 유저의 id와 queue에 유저와 쿠폰 정보를 넣습니다. consumer에서 queue의 데이터를 가져 간후 redis에서 해당 데이터를 삭제하기 위해 removeOnComplete를 추가하였습니다.
saveCouponIssue = async (params: { userId: number; couponId: number }) => {
await this.redis.sadd(this.couponSet, params.userId);
(await BullMq.getBullMq()).add(
this.couponQueue,
{ userId: params.userId, couponId: params.couponId },
{
removeOnComplete: true,
}
);
};
기존에 mysql을 사용하여 쿠폰발급을 하였을 때, RPS가 135였는데 redis를 사용하니 RPS가 827까지 올랐습니다. 대략 5~6배의 성능 차이를 보이고 있습니다.
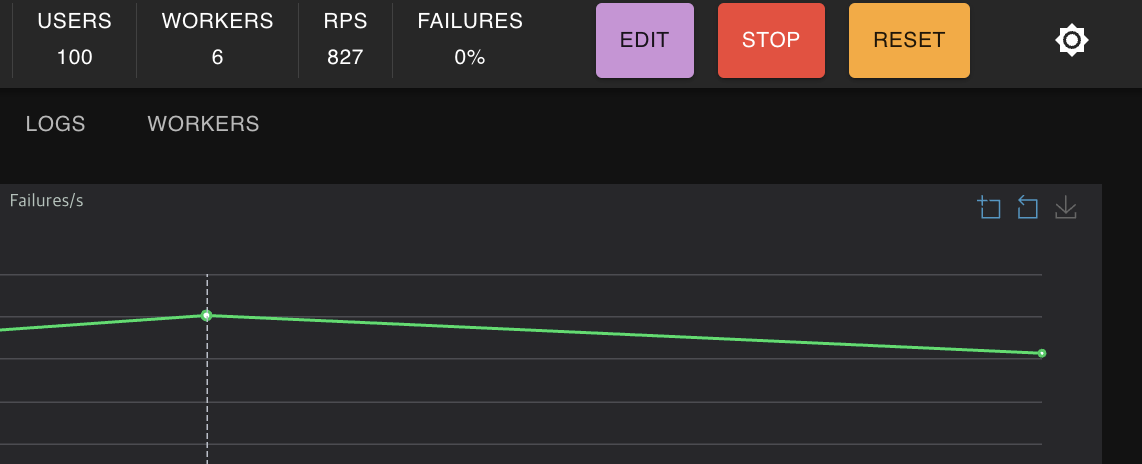

하지만 쿠폰은 최대 발급 수량이 10000개보다, 58개 더 발급 되었습니다. 이는 mysql와 같은 문제로 redis는 single thead로 처리하지만, redis로 요청을 보내는 로직에서 동시성 제어가 처리되지 않아서 발생하는 문제입니다.
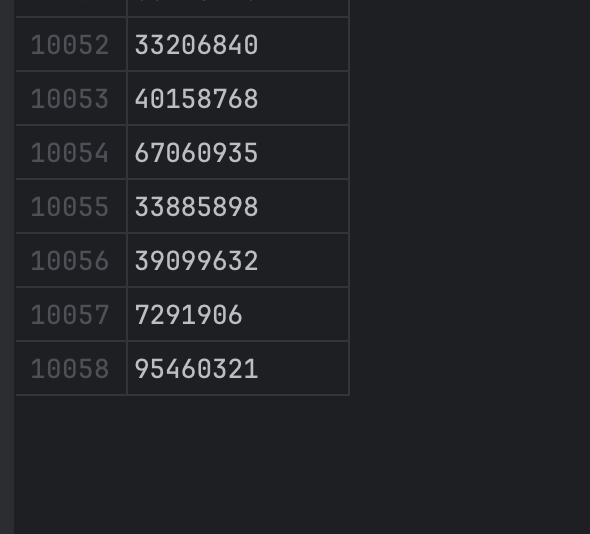
마찬가지로 redis 쿠포발급하는 임계영역도 동시성 처리를 해줍니다.
app.post("/issueCouponV2", async (req: any, res: any, next) => {
const mutex = new Mutex(await Redis.getRedisClient(), "couponKey", {
acquireTimeout: 100000,
});
try {
const redisCouponService = new RedisCouponIssueService(
await Redis.getRedisClient()
);
await mutex.acquire();
await redisCouponService.issueCoupon({
userId: req.body.userId,
couponId: req.body.couponId,
});
return res.status(200).json({ message: "쿠폰 발급 성공" });
} catch (err) {
return next(err);
} finally {
await mutex.release();
}
});
확실히 동시성 제어를 하니, RPS가 827 -> 179로 떨어진 것을 확인할 수 있습니다. 마찬가지로 RPS가 떨어지니, redsi cpu 사용량도 줄었습니다.
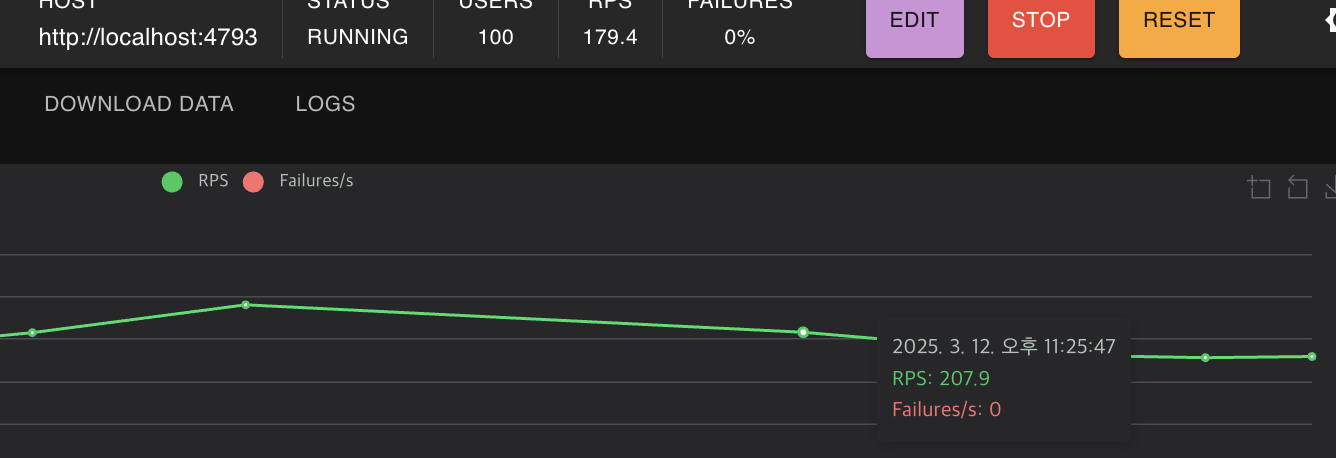

기존 mysql 쿠폰 발급의 RPS 135에 비해 34정도 향상된 모습을 볼 수 있습니다.
쿠폰 발급 서버에서 queue에 쿠폰을 삽입하면 쿠폰 발행 서버에서는 queue에 있는 데이터를 읽어와 db에 삽입할 수 있습니다. 실제 쿠폰을 발행하는 서버는 백그라운드로 돌리고 쿠폰 발급서버는 클라이언트의 요청을 처리함으로써, 쿠폰을 발급하는 서버의 부하를 분산시킬 수 있습니다.
import { Queue, Worker } from "bullmq";
import IORedis from "ioredis";
import mysql from "mysql2/promise";
(async () => {
const redis = new IORedis({
host: "localhost",
port: 6379,
maxLoadingRetryTime: null,
maxRetriesPerRequest: null,
});
new Worker(
"Coupon",
async (job) => {
const connection = await mysql.createConnection({
host: "localhost",
user: "root",
port: 3306,
password: "xxxx",
database: "xxxx",
});
const data = job.data as { userId: number; couponId: number };
await connection.execute(
`insert into CouponIssue(couponId, userId) values(${data.couponId}, ${data.userId})`
);
await connection.end();
},
{ connection: redis }
);
})();
'개발' 카테고리의 다른 글
promise pool로 비동기 작업 처리량 높이기 (0) | 2025.04.03 |
---|---|
monorepo프로젝트 docker build하기 (0) | 2025.02.27 |
git action에서 Cloud SQL 인증 프록시로 db migration 하기 (0) | 2025.02.13 |
[Redis] 레디스 기본타입(Sorted Sets) (0) | 2024.03.30 |
[Redis] 레디스 기본타입(Sets) (0) | 2024.02.12 |